Java I/O Tutorial
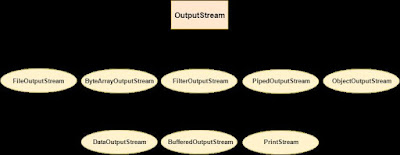
Java I/O Tutorial Java I/O (Input and Output) is used to process the input and produce the output . Java uses the concept of stream to make I/O operation fast. The java.io package contains all the classes required for input and output operations. We can perform file handling in java by Java I/O API. Stream A stream is a sequence of data. In Java a stream is composed of bytes. It's called a stream because it is like a stream of water that continues to flow. In java, 3 streams are created for us automatically. All these streams are attached with console. 1) System.out: standard output stream 2) System.in: standard input stream 3) System.err: standard error stream Let's see the code to print output and error message to the console. System.out.println( "simple message" ); System.err.println( "error message" ); Let's see the code to get input from console. int i=System.in.read(); //returns AS